Post-Order Traversal of a Tree
Given an array of integers. Create a Binary Search Tree from these numbers. If the inserted value equals to the current node, insert it to the right subtree.
Write method PostOrder
that makes Post-Order traversal of a tree. In this traversal method the left subtree is visited first, then the right subtree and finally the root node.
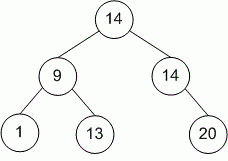
Write the code according to the next interface:
class TreeNode
{
public:
int val;
TreeNode *left;
TreeNode *right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
class Tree
{
public:
TreeNode *head;
Tree() : head(NULL) {};
void Insert(int val); // Insert number val to Binary Search Tree
void PostOrder(void); // Print the vertices of a tree according to post-order traversal
};
You can create (use) additional methods if needed.
Input
The first line contains number n (1 ≤ n ≤ 100). The second line contains n integers.
Output
Create the Binary Search Tree from input data. Print the vertices of a tree according to post-order traversal.