Algorithm Analysis
The number of leaves to the right of the stem equals (the tree contains tiers). To the left of the stem, the number of leaves is the same. And one more leaf on top.
The number of liters of water for irrigation equals the number of leaves in the tree. It equals
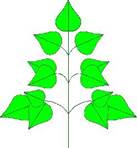
Algorithm Implementation
Let's perform the calculation according to the formula mentioned above.
scanf("%d",&n); res = n * (n + 1) + 1; printf("%d\n",res);
Implementation via Pointers
#include <stdio.h> int *n, *res; int main(void) { n = new int; res = new int; scanf("%d",n); *res = *n * (*n + 1) + 1; printf("%d\n",*res); delete n; delete res; return 0; }
Implementation Using a Class
#include <stdio.h> class Long { private: long long value; public: Long(long long value = 0) : value(value) {} void Read(void) { scanf("%lld",&value); } void Print(void) { printf("%lld\n",value); } Long operator+ (long long x) { return value + x; } Long operator* (const Long &x) { return value * x.value; } }; int main(void) { Long n, res; n.Read(); res = n * (n + 1) + 1; res.Print(); return 0; }
Java Implementation
import java.util.*; public class Main { public static void main(String[] args) { Scanner con = new Scanner(System.in); int n = con.nextInt(); int res = n * (n + 1) + 1; System.out.println(res); } }
Python Implementation
n = int(input()) res = n * (n + 1) + 1 print(res)