Editorial
Each query can be performed using a loop with a complexity of . We have queries and the length of the array is . If each query runs in linear time, we would need no more than operations, which will exceed the Time Limit.
Consider the partial sums of array :
It is possible to compute the values in a linear array in time.
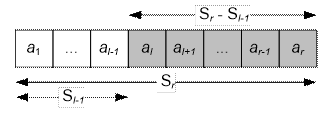
Further note that
So we can answer the query in constant time.
Example
Let's compute the sum for the input example.
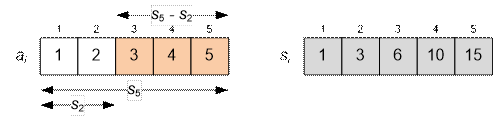
We have:
Algorithm realization
Declare two working two-dimensional arrays.
#define MAX 1000001 int a[MAX], s[MAX];
Read the input set of numbers into array , and calculate the partial sums in array .
scanf("%d",&n); s[0] = 0; for(i = 1; i <= n; i++) { scanf("%d",&a[i]); s[i] = s[i-1] + a[i]; }
Process queries.
scanf("%d",&m); for(i = 0; i < m; i++) { scanf("%d %d",&b,&c); printf("%d\n",s[c] - s[b-1]); }
Python realization
Read the input data.
n = int(input()) a = [0] + list(map(int, input().split()))
Calculate the partial sums of list in list .
s = [0] * (n + 1) for i in range(1, n + 1): s[i] = s[i - 1] + a[i]
Process queries.
m = int(input()) for _ in range(m): b, c = map(int, input().split()) print(s[c] - s[b - 1])